Create Remote Functions using Amazon AWS Lambda
Create a Java project
We will create a maven based Java project. This project needs to define a method which can be called AWS Lambda function. To keep things simple, we will declare a method which returns "Welcome to Gluon AWS Lambda Demo".
package com.gluonhq;
public class GCLHandler {
public String handleRequest() {
return "Welcome to Gluon AWS Lambda Demo";
}
}
The pom.xml
for the project contains the maven-compiler-plugin
to set the Java version to 1.8.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.gluonhq</groupId>
<artifactId>aws-lambda-gcl</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.7.0</version>
</plugin>
</plugins>
</build>
</project>
Once we are done with the project creation, we can fire the following command to create a jar in the target directory of the project.
mvn clean package
This jar can be uploaded to the AWS lambda function.
Create an AWS Lambda Function
In order to create an AWS lambda function, log in to the AWS Console Management using your IAM user console login link and create a lambda function for your choice of runtime. In our case, we will use a Java 8 runtime.
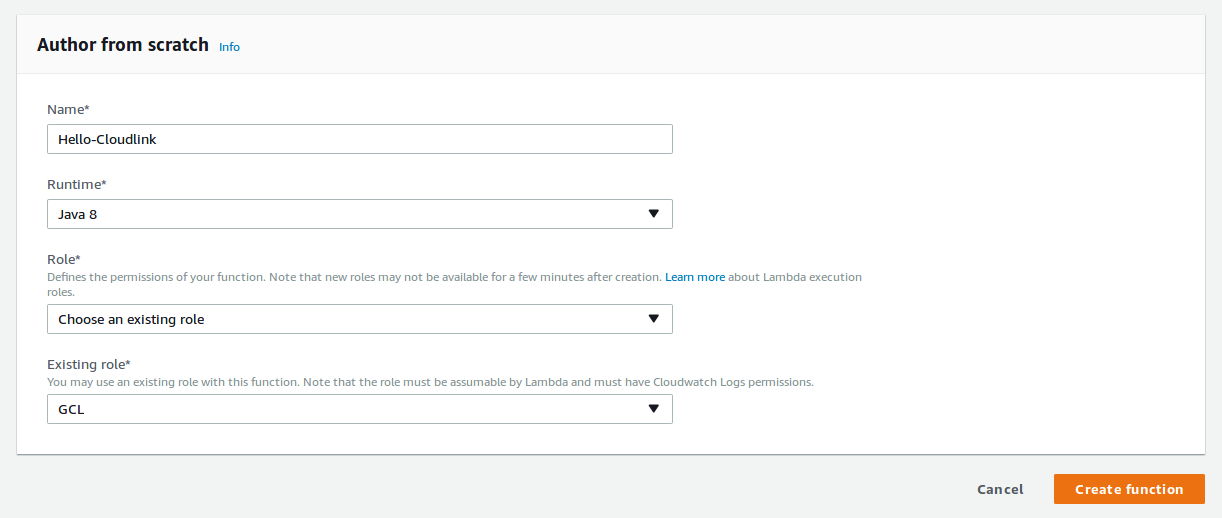
Scroll down to the section "Function code". Update the Handler info with the method name. This is the complete
name of the class followed by the method which needs to be executed. In our case it is com.gluonhq.GCLHandler::handleRequest
.
Next, we upload the jar that was created in the previous step.
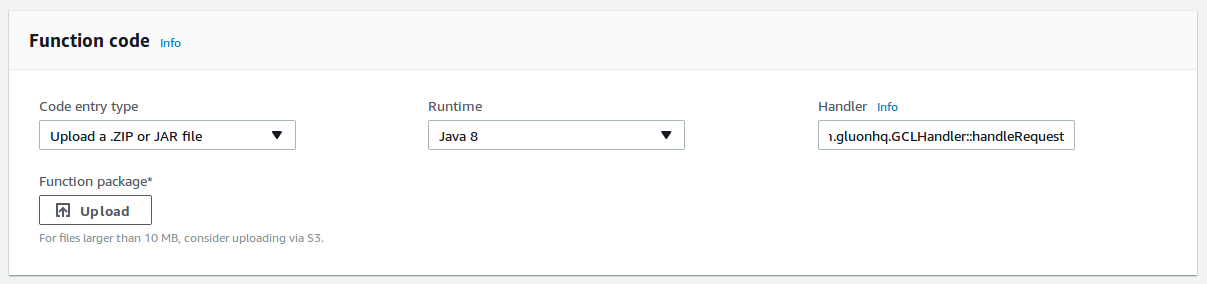
We can now save this and move to the Gluon CloudLink dashboard.
Setting up Gluon CloudLink
Before you can create a remote function for an AWS Lambda function, you need to add customer credentials that point to a valid Amazon AWS access key. This has been explained in details in our AWS Lamda documentation.
For this example, we have setup the credentials for AWS with name "AWS Access". Navigate back to the API Management section and click the + button to add a new remote function while choosing Amazon AWS Lambda. A dialog will be shown where the AWS credential along with the AWS lambda function, created earlier, can be chosen.
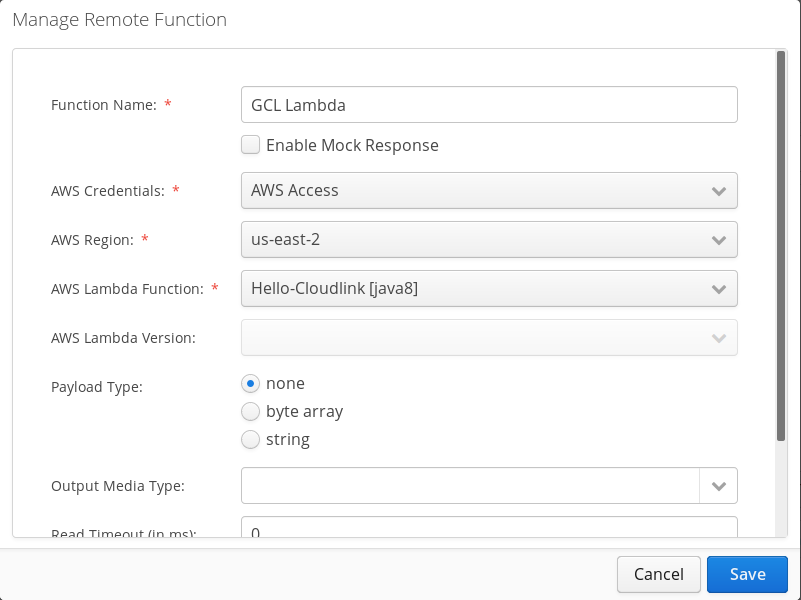
Testing the setup in Gluon CloudLink
We should now be able to invoke the AWS Lambda function using our Gluon CloudLink dashboard. In order to do this, we can simply choose the function from the drop down available besides the "+" button. The details of the remote function is displayed.
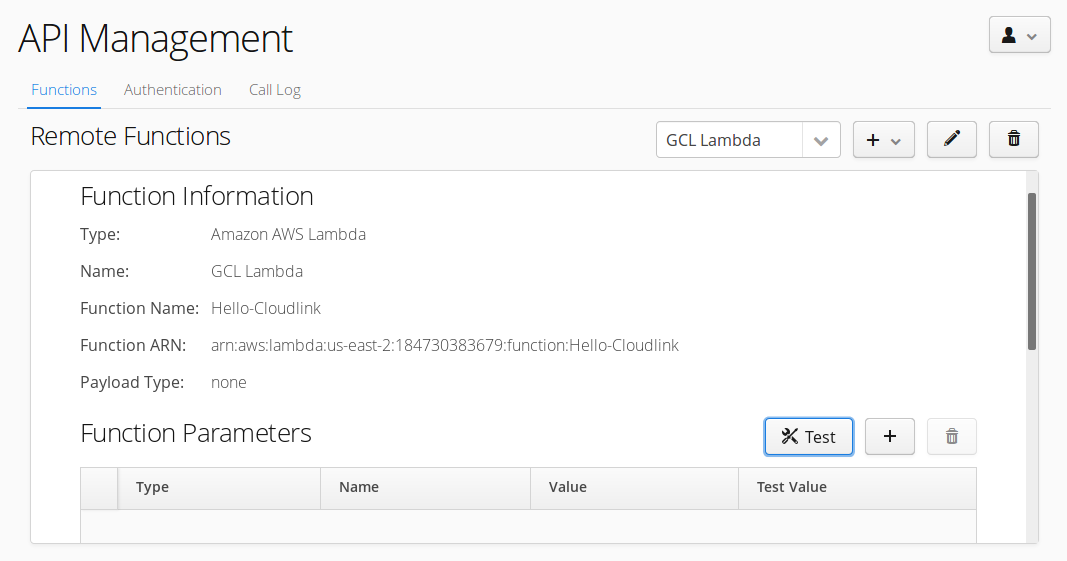
We can now create the function parameters that we want to pass to our function. Since, our remote function is very basic, it doesn’t have any parameters (For more complex examples, please check the links at the bottom of the documentation). We can click on "Test". It will display the string "Welcome to Gluon AWS Lambda Demo" in a dialog.
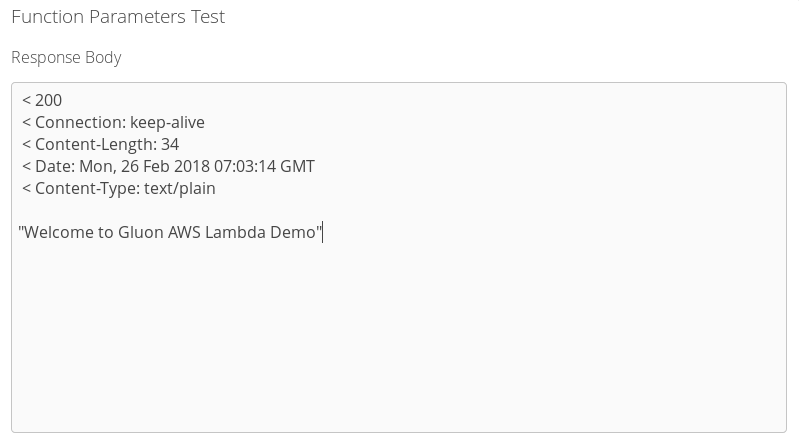
For calling the remote function from your application, please refer to CloudLink Client section.
Some useful links: